Exiftool Python
A command-line interface to Image::ExifTool, used for reading and writing meta information in image, audio and video files. FILE is a source file name, directory name, or -for the standard input. Information is read from the source file and output in readable form to the console (or written to an output text file with the -w option). To write or copy information, new values are. ExifTool supports JSON output, which is probably the best option for reading the metadata. Here's a simple class that launches an exiftool process and features an execute method to send commands to that process. I also included getmetadata to read the metadata in JSON format. Alternatively, you can run exiftool with the command 'perl exiftool'. IF YOU ARE STILL CONFUSED The exiftool script is a command line application. You run it by typing commands in a terminal window. The first step is to determine the name of the directory where you downloaded the ExifTool distribution package. Exiftool is a mature and reliable metadata reading and writing application. Although this article was written in 2010, Exiftool and the Exiftool syntax seldom change except to add new tags and new capabilities. I put this article up on my website mostly so I would have a quick reference for the Exiftool commands that I use the most often.
PyExifTool is a Python library to communicate with an instance of PhilHarvey’s excellent ExifTool command-line application. The libraryprovides the class ExifTool that runs the command-linetool in batch mode and features methods to send commands to thatprogram, including methods to extract meta-information from one ormore image files. Since exiftool is run in batch mode, only asingle instance needs to be launched and can be reused for manyqueries. This is much more efficient than launching a separateprocess for every single query.
The source code can be checked out from the github repository with
Alternatively, you can download a tarball. There haven’t been anyreleases yet.
PyExifTool is licenced under GNU GPL version 3 or later.
Example usage:
Run the exiftool command-line tool and communicate to it.
You can pass the file name of the exiftool executable as anargument to the constructor. The default value exiftool willonly work if the executable is in your PATH.
Most methods of this class are only available after callingstart(), which will actually launch the subprocess. Toavoid leaving the subprocess running, make sure to callterminate() method when finished using the instance.This method will also be implicitly called when the instance isgarbage collected, but there are circumstance when this won’t everhappen, so you should not rely on the implicit processtermination. Subprocesses won’t be automatically terminated ifthe parent process exits, so a leaked subprocess will stay arounduntil manually killed.
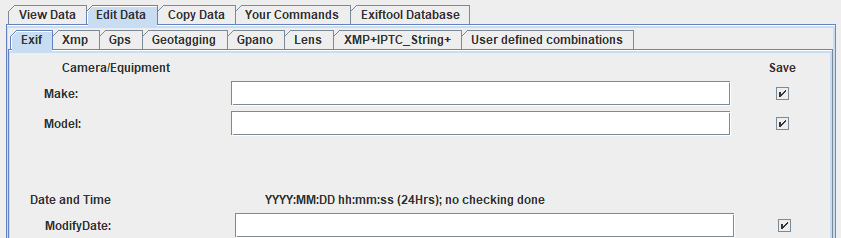
A convenient way to make sure that the subprocess is terminated isto use the ExifTool instance as a context manager:
Warning
Note that there is no error handling. Nonsensicaloptions will be silently ignored by exiftool, so there’s notmuch that can be done in that regard. You should avoid passingnon-existent files to any of the methods, since this will leadto undefied behaviour.
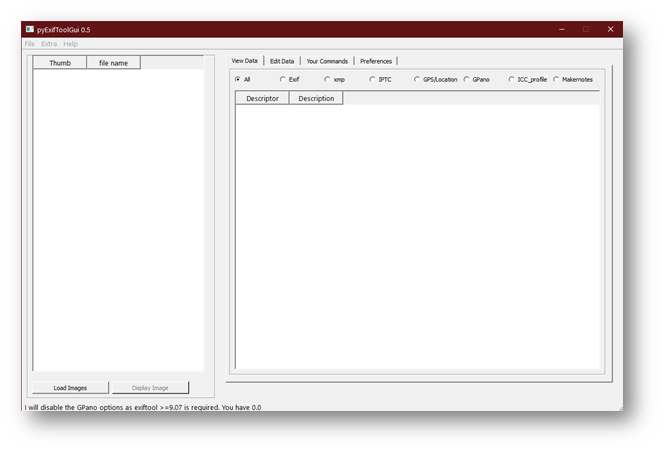
A Boolean value indicating whether this instance is currentlyassociated with a running subprocess.
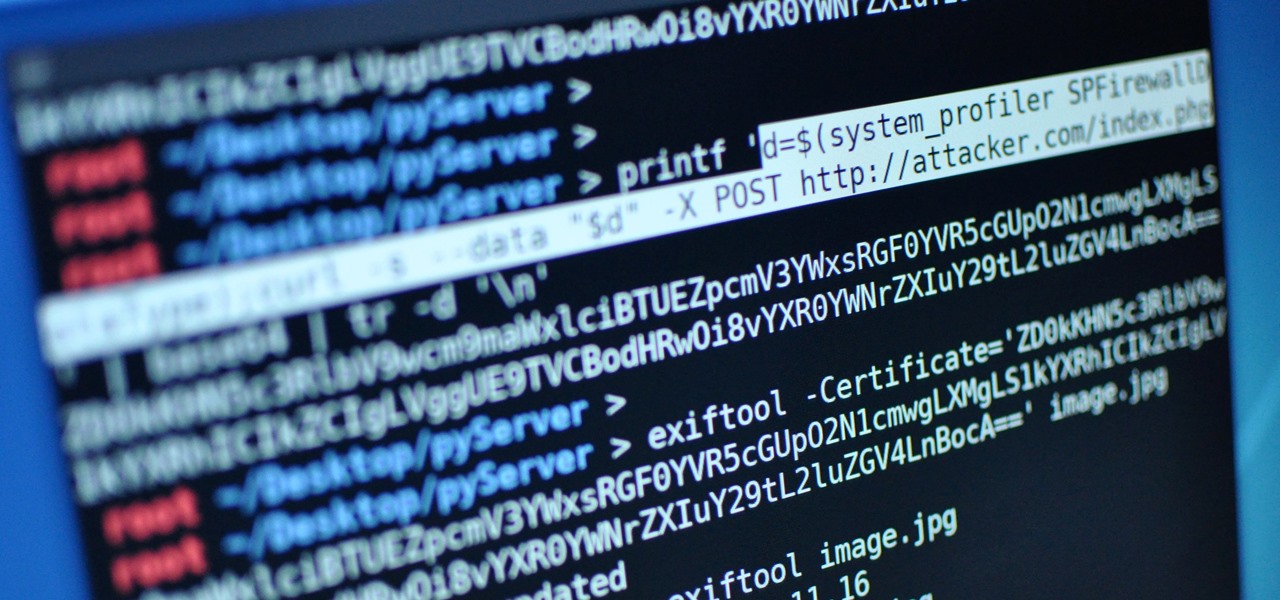
Execute the given batch of parameters with exiftool.
Exiftool Python
This method accepts any number of parameters and sends them tothe attached exiftool process. The process must berunning, otherwise ValueError is raised. The final-execute necessary to actually run the batch is appendedautomatically; see the documentation of start() forthe common options. The exiftool output is read up to theend-of-output sentinel and returned as a raw bytes object,excluding the sentinel.
The parameters must also be raw bytes, in whateverencoding exiftool accepts. For filenames, this should be thesystem’s filesystem encoding.
Note
This is considered a low-level method, and shouldrarely be needed by application developers.
Execute the given batch of parameters and parse the JSON output.
This method is similar to execute(). Itautomatically adds the parameter -j to request JSON outputfrom exiftool and parses the output. The return value isa list of dictionaries, mapping tag names to the correspondingvalues. All keys are Unicode strings with the tag names,including the ExifTool group name in the format <group>:<tag>.The values can have multiple types. All strings occurring asvalues will be Unicode strings.
The parameters to this function must be either raw strings(type str in Python 2.x, type bytes in Python 3.x) orUnicode strings (type unicode in Python 2.x, type strin Python 3.x). Unicode strings will be encoded usingsystem’s filesystem encoding. This behaviour means you canpass in filenames according to the convention of therespective Python version – as raw strings in Python 2.x andas Unicode strings in Python 3.x.
Exiftool Python Commands
Return meta-data for a single file.
The returned dictionary has the format described in thedocumentation of execute_json().
Return all meta-data for the given files.
The return value will have the format described in thedocumentation of execute_json().
Extract a single tag from a single file.
The return value is the value of the specified tag, orNone if this tag was not found in the file.
Extract a single tag from the given files.
The first argument is a single tag name, as usual in theformat <group>:<tag>.
The second argument is an iterable of file names.

The return value is a list of tag values or None fornon-existent tags, in the same order as filenames.
Return only specified tags for a single file.
The returned dictionary has the format described in thedocumentation of execute_json().
PyExifToolGUI - GitHub Pages
Return only specified tags for the given files.
The first argument is an iterable of tags. The tag names mayinclude group names, as usual in the format <group>:<tag>.
The second argument is an iterable of file names.
The format of the return value is the same as forexecute_json().
Start an exiftool process in batch mode for this instance.
This method will issue a UserWarning if the subprocess isalready running. The process is started with the -G and-n as common arguments, which are automatically includedin every command you run with execute().
Terminate the exiftool process of this instance.
If the subprocess isn’t running, this method will do nothing.
The name of the executable to run.
If the executable is not located in one of the paths listed in thePATH environment variable, the full path should be given here.
Encode filename to the filesystem encoding with ‘surrogateescape’ errorhandler, return bytes unchanged. On Windows, use ‘strict’ error handler ifthe file system encoding is ‘mbcs’ (which is the default encoding).
